Font Functions
HPDF_Font_GetFontName
#include
"apdf.h"
const char *
HPDF_Font_GetFontName (HPDF_Font font);
const char *
HPDF_Font_GetFontName (HPDF_Font font);
Description
HPDF_Font_GetFontName() gets the name of the font.Parameter
fontThe handle of an font object.
Returns
When HPDF_Font_GetFontName() succeed, it returns the name of the font. Otherwise, it returns NULL.HPDF_Font_GetEncodingName
#include
"apdf.h"
const char *
HPDF_Font_GetEncodingName (HPDF_Font font);
const char *
HPDF_Font_GetEncodingName (HPDF_Font font);
Description
HPDF_Font_GetEncodingName() gets the encoding name of the font.Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetEncodingName() succeed, it returns the encoding name of the font. Otherwise, it returns NULL.HPDF_Font_GetUnicodeWidth
#include
"apdf.h"
HPDF_INT
HPDF_Font_GetUnicodeWidth (HPDF_Font font,
HPDF_UNICODE code);
HPDF_INT
HPDF_Font_GetUnicodeWidth (HPDF_Font font,
HPDF_UNICODE code);
Description
HPDF_Font_GetUnicodeWidth() gets the width of a charactor in the font.Actual width of the character on the page can be calculated by the following
expressions.
char_width = HPDF_Font_GetUnicodeWidth (font, UNICODE);
float actual_width = char_width * FONT_SIZE / 1000;
Parameter
fontThe handle of an font object.
code
A unicode of the charactor.
Returns
When HPDF_Font_GetUnicodeWidth() succeed, it returns the width of the font. Otherwise it returns 0.HPDF_Font_GetBBox
#include
"apdf.h"
typedef struct _HPDF_Rect {
HPDF_REAL left;
HPDF_REAL bottom;
HPDF_REAL right;
HPDF_REAL top;
} HPDF_Rect;
typedef struct _HPDF_Rect HPDF_Box;
HPDF_Box
HPDF_Font_GetBBox (HPDF_Font font);
typedef struct _HPDF_Rect {
HPDF_REAL left;
HPDF_REAL bottom;
HPDF_REAL right;
HPDF_REAL top;
} HPDF_Rect;
typedef struct _HPDF_Rect HPDF_Box;
HPDF_Box
HPDF_Font_GetBBox (HPDF_Font font);
Description
HPDF_Font_GetBBox() gets the bounding box of the font.Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetBox() succeed, it returns the HPDF_Box struct specifying the font bounding box. Otherwise, it returns a HPDF_Box struct of {0, 0, 0, 0}.HPDF_Font_GetAscent
#include
"apdf.h"
HPDF_INT
HPDF_Font_GetAscent (HPDF_Font font);
HPDF_INT
HPDF_Font_GetAscent (HPDF_Font font);
Description
HPDF_Font_GetAscent() gets the vertical ascent of the font. Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetAscent() succeed, it returns vertical ascent of the font. Otherwise, it returns 0.HPDF_Font_GetDescent
#include
"apdf.h"
HPDF_INT
HPDF_Font_GetDescent (HPDF_Font font);
HPDF_INT
HPDF_Font_GetDescent (HPDF_Font font);
Description
HPDF_Font_GetDescent() gets the vertical descent of the font. Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetDescent() succeed, it returns vertical descent of the font. Otherwise, it returns 0.HPDF_Font_GetXHeight
#include
"apdf.h"
HPDF_UINT
HPDF_Font_GetXHeight (HPDF_Font font);
HPDF_UINT
HPDF_Font_GetXHeight (HPDF_Font font);
Description
HPDF_Font_GetXHeight() gets the distance from the baseline of lowercase
letters. Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetXHeight() succeed, it returns the x-height's value of the font. Otherwise, it returns 0.HPDF_Font_GetCapHeight
#include
"apdf.h"
HPDF_UINT
HPDF_Font_GetCapHeight (HPDF_Font font);
HPDF_UINT
HPDF_Font_GetCapHeight (HPDF_Font font);
Description
HPDF_Font_GetCapHeight() gets the distance from the baseline of
uppercase letters. Parameter
fontThe handle of a font object.
Returns
When HPDF_Font_GetCapHeight() succeed, it returns the cap-height's value of the font. Otherwise, it returns 0.HPDF_Font_TextWidth
#include
"apdf.h"
typedef struct _HPDF_TextWidth {
HPDF_UINT numchars;
HPDF_UINT numwords;
HPDF_UINT width;
HPDF_UINT numspace;
} HPDF_TextWidth;
HPDF_TextWidth
HPDF_Font_TextWidth (HPDF_Font font,
const HPDF_BYTE *text,
HPDF_UINT len);
typedef struct _HPDF_TextWidth {
HPDF_UINT numchars;
HPDF_UINT numwords;
HPDF_UINT width;
HPDF_UINT numspace;
} HPDF_TextWidth;
HPDF_TextWidth
HPDF_Font_TextWidth (HPDF_Font font,
const HPDF_BYTE *text,
HPDF_UINT len);
Description
HPDF_Font_TextWidth() gets total width of the text, number of
charactors and number of the words. Parameter
fontThe handle of a font object.
text
The text to get width.
len
The byte length of the text.
Returns
When HPDF_Font_TextWidth() succeed, it returns a HPDF_TextWidth struct including calculation result. Otherwise, it returns a HPDF_TextWidth struct whose attributes are all ZERO.HPDF_Font_MeasureText
#include
"apdf.h"
HPDF_UINT
HPDF_Font_MeasureText (HPDF_Font font,
const HPDF_BYTE *text,
HPDF_UINT len,
HPDF_REAL width,
HPDF_REAL font_size,
HPDF_REAL char_space,
HPDF_REAL word_space,
HPDF_BOOL wordwrap,
HPDF_REAL *real_width);
HPDF_UINT
HPDF_Font_MeasureText (HPDF_Font font,
const HPDF_BYTE *text,
HPDF_UINT len,
HPDF_REAL width,
HPDF_REAL font_size,
HPDF_REAL char_space,
HPDF_REAL word_space,
HPDF_BOOL wordwrap,
HPDF_REAL *real_width);
Description
HPDF_Font_MeasureText() calculates the byte length which can be
included within the specified width.Parameter
fontSpecify the handle of a font object.
text
A text that is used to calculate.
lenThe length of the text.
widthThe width of the area to put the text.
font_sizeThe size of the font.
char_spaceThe character spacing.
word_spaceThe word spacing.
wordwrapWhen there are three words of "ABCDE", "FGH",
and "IJKL", and the substring until "J" can be included within the
width, if word_wrap parameter is HPDF_FALSE it returns 12, and if
word_wrap parameter is HPDF_FALSE word_wrap parameter is HPDF_FALSE it
returns 10 (the end of the previous word).
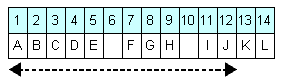
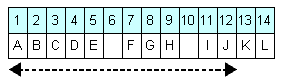
real_width
If this parameter is not NULL, the real widths
of the text is set. An application can set it to NULL if it is
unnecessary