How to use
Overview
The typical program flow of Haru is as
follows.
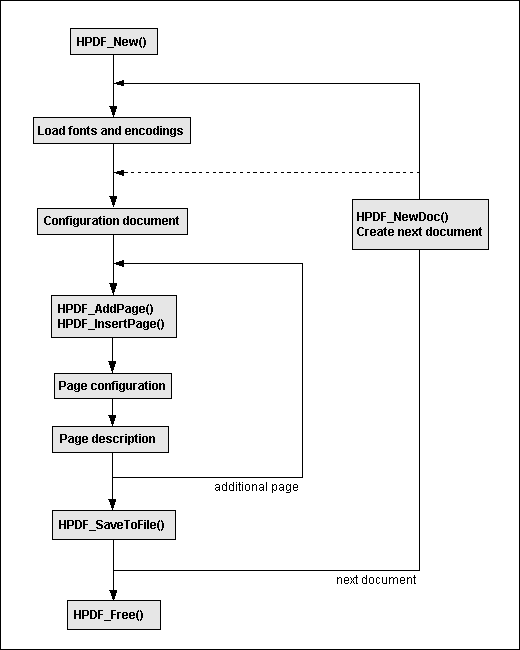
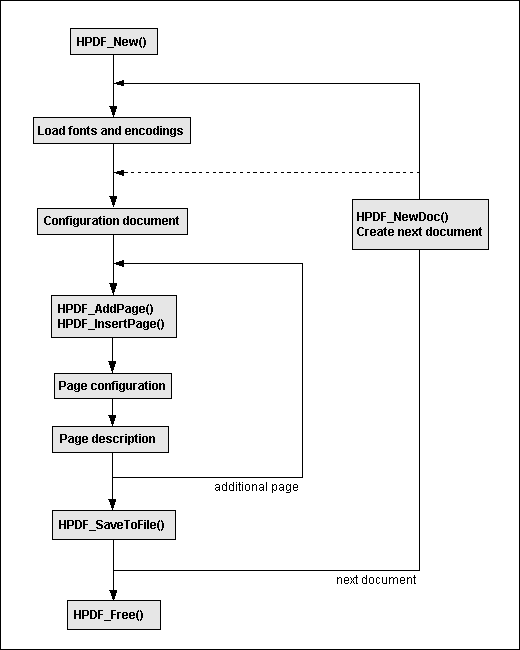
Initializing a
document object
First, invoke HPDF_New() or HPDF_NewEx() to
create
an instance of document object.
You can specify user-defined error function on this step. If you want to use user-define memory memory management function, use HPDF_NewEx().
The Handle which is returned from HPDF_New() or HPDF_NewEx() is used in the following steps.
You can call setjmp() for an exception handling after HPDF_New() succeed. (See the chapter of "Error Handling")
You can specify user-defined error function on this step. If you want to use user-define memory memory management function, use HPDF_NewEx().
The Handle which is returned from HPDF_New() or HPDF_NewEx() is used in the following steps.
You can call setjmp() for an exception handling after HPDF_New() succeed. (See the chapter of "Error Handling")
#include "apdf.h"
HPDF_Doc pdf;
pdf = HPDF_New (error_handler, NULL);
if (!pdf) {
printf ("ERROR: cannot create pdf object.\n");
return 1;
}
if (setjmp(env)) {
HPDF_Free (pdf);
return 1;
}
HPDF_Doc pdf;
pdf = HPDF_New (error_handler, NULL);
if (!pdf) {
printf ("ERROR: cannot create pdf object.\n");
return 1;
}
if (setjmp(env)) {
HPDF_Free (pdf);
return 1;
}
Setting a document
object
Set attributes of compression, encryption,
page mode and pages tree if necessary.
/* set compression mode */
HPDF_SetCompressionMode (pdf, HPDF_COMP_ALL);
/* set page mode to use outlines. */
HPDF_SetPageMode (pdf, HPDF_PAGE_MODE_USE_OUTLINE);
/* set password */
HPDF_SetPassword (pdf, "owner", "user");
HPDF_SetCompressionMode (pdf, HPDF_COMP_ALL);
/* set page mode to use outlines. */
HPDF_SetPageMode (pdf, HPDF_PAGE_MODE_USE_OUTLINE);
/* set password */
HPDF_SetPassword (pdf, "owner", "user");
Create a page
object
Call HPDF_AddPage() to add a
page to the
document. The page-handle which is returned by HPDF_AddPage() function
is used to operate a page.
page_1 = HPDF_AddPage (pdf);
When you want to insert a new page before an
existing page, call HPDF_InsertPage().
For example, in the case of
inserting "page_0" before "page_1", the code is as follows.
page_0 = HPDF_InsertPage (pdf, page_1);
Setting a page object
Set attributes of the page object if necessary.
HPDF_Page_SetSize (page_1,
HPDF_PAGE_SIZE_B5, HPDF_PAGE_LANDSCAPE);
Page description
You can perform drawing processing for a page.
For details, please refer to a chapter of "Graphics".
Save a document to file or
memory stream.
Call HPDF_SaveToFile()
to save a document to a
file.
If you don't want to save a document to a file, use HPDF_SaveToStream() instead. HPDF_SaveToStream() saves a document to a temporary stream.
An application can get the data saved by HPDF_SaveToStream() by invoking HPDF_ReadFromStream().
The following codes are examples of outputting the document directly to stdout.
/* save the document to a stream */
HPDF_SaveToStream (pdf);
fprintf (stderr, "the size of data is %d\n", HPDF_GetStreamSize(pdf));
/* rewind the stream. */
HPDF_ResetStream (pdf);
/* get the data from the stream and output it to stdout. */
for (;;) {
HPDF_BYTE buf[4096];
HPDF_UINT32 siz = 4096;
HPDF_STATUS ret = HPDF_ReadFromStream (pdf, buf, &siz);
if (siz == 0)
break;
if (fwrite (buf, siz, 1, stdout) != 1) {
fprintf (stderr, "cannot write to stdout", siz, wsiz);
break;
}
}
/* save the document to a file */
HPDF_SaveToFile (pdf, "test.pdf");
HPDF_SaveToFile (pdf, "test.pdf");
If you don't want to save a document to a file, use HPDF_SaveToStream() instead. HPDF_SaveToStream() saves a document to a temporary stream.
An application can get the data saved by HPDF_SaveToStream() by invoking HPDF_ReadFromStream().
The following codes are examples of outputting the document directly to stdout.
/* save the document to a stream */
HPDF_SaveToStream (pdf);
fprintf (stderr, "the size of data is %d\n", HPDF_GetStreamSize(pdf));
/* rewind the stream. */
HPDF_ResetStream (pdf);
/* get the data from the stream and output it to stdout. */
for (;;) {
HPDF_BYTE buf[4096];
HPDF_UINT32 siz = 4096;
HPDF_STATUS ret = HPDF_ReadFromStream (pdf, buf, &siz);
if (siz == 0)
break;
if (fwrite (buf, siz, 1, stdout) != 1) {
fprintf (stderr, "cannot write to stdout", siz, wsiz);
break;
}
}
Create next document
If you want to create next document, call
HPDF_NewDoc().
HPDF_NewDoc() create a new document after revoking a current document.
HPDF_NewDoc() create a new document after revoking a current document.
HPDF_NewDoc (pdf);
Finalization and cleanup
After all processes are finished, call
HPDF_Free() to free
all resources which belong to the document object.
/* cleanup */
HPDF_Free (pdf);
HPDF_Free (pdf);